Developing games in Java has become a popular choice for many aspiring game developers. Known for its versatility, ease of learning, and extensive libraries, Java provides a great environment to build 2D and 3D games – but does one learn how to develop games in Java, exactly?
Whether you are new to programming or an experienced coder, Java offers powerful tools and frameworks to help you create interactive and visually appealing games.
In this article, we want to explore the key steps and considerations for the development of games in Java. Our goal is to cover everything from the basics of setting up your environment to building your first game, with some examples of codes along the way!
You may also like: What is game development? A quick guide to beginners
How to develop games in Java: why choose Java?
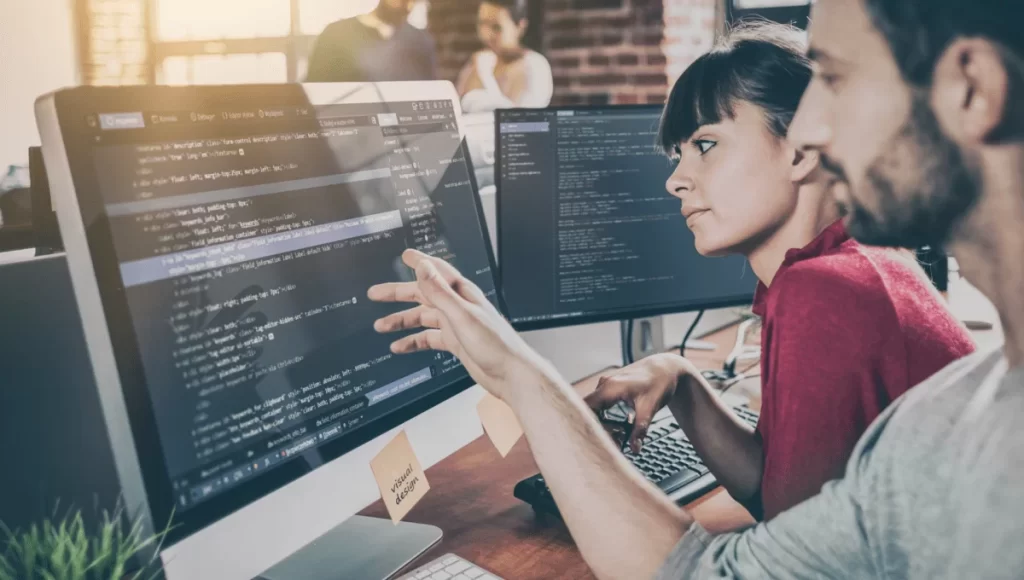
Before diving into the details of developing games in Java, it’s important to understand why Java is a solid choice for game development, and this can be broken down into three simple points.
1: Cross-platform compatibility
Java is platform-independent due to its “write once, run anywhere” philosophy. This makes it easier to deploy games across different operating systems, including Windows, macOS, and Linux.
2: Large community and support
Java has been around for decades, which means there is a large community of developers who have contributed to its growth. This translates into vast resources, tutorials, and libraries available to support your game development journey.
3: Rich libraries and frameworks
A big reason why how to develop games in Java is because it provides several game development frameworks, such as LibGDX and Slick2D. These frameworks simplify the process of creating 2D and 3D games by handling graphics, physics, and other key components.
How to develop games in Java in 5 steps
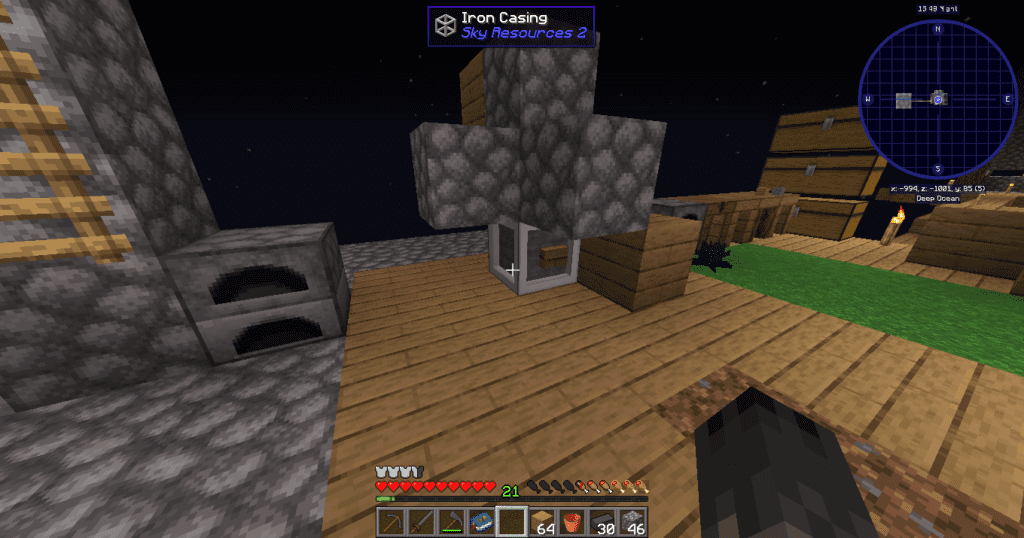
Step 1: setting up your development environment
To start developing games in Java, you’ll need to set up a development environment. A development environment in Java refers to the set of tools and software used to create, test, and run Java programs. It includes everything a developer needs to write and execute code effectively.
First, you need to install the Java Development Kit (JDK). The JDK is essential for writing and running Java applications. You can download it from the official Oracle website.
Next, you choose an Integrated Development Environment (IDE). Popular IDEs like IntelliJ IDEA, Eclipse, or NetBeans are commonly used for Java development. These IDEs offer tools for debugging, code completion, and project management, making your workflow smoother.
And then you need to install Game Development Libraries. Depending on the type of game you want to develop, you’ll need to install the appropriate libraries or frameworks, such as the aforementioned LibGDX or Slick2D.
Step 2: learn the basics of Java programming
A big part of how to develop games in Java is, of course, to know its coding language. If you’re new to Java, you’ll need to familiarize yourself with the basics of the language. Here are a few key concepts to focus on:
- Object-oriented programming (OOP): Java is an object-oriented language, which means it uses classes and objects to structure code. You’ll need to understand how to create classes, use inheritance, and implement polymorphism to build efficient game mechanics.
- Loops and conditionals: Loops (for, while) and conditionals (if-else, switch) are essential for controlling game logic, such as managing player actions and game states.
- Graphics and event handling: For games, you’ll work extensively with graphics (drawing shapes, sprites) and handling user input (keyboard, mouse). Java’s java.awt and javax.swing libraries are useful for creating basic game interfaces and handling events.
Step 3: start building your first game
Once you’ve set up your environment and learned the basics of Java, you’re ready to start building a game.
Start with a simple game idea, like a classic arcade game or a basic puzzle. Planning involves defining your game mechanics, player controls, levels, and objectives. Sketch out how the game will look, and consider what resources you’ll need, such as images for characters and backgrounds.
Read also: Just how long does it take to make a game?
With an idea in mind, now you need to set up your game window. In Java, you can create a game window using the JFrame class from the javax.swing package. This window will serve as the main canvas where your game will run. You’ll also need to use JPanel to manage the content inside the window.
import javax.swing.JFrame;
import javax.swing.JPanel;
public class GameWindow extends JFrame {
public GameWindow() {
setTitle("My First Game");
setSize(800, 600);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
}
public static void main(String[] args) {
GameWindow window = new GameWindow();
window.setVisible(true);
}
}
Once you have your window set up, the next step is to add game mechanics. This includes defining player controls, game physics, and rendering objects. A basic 2D game, for example, might involve moving a character on the screen using arrow keys.
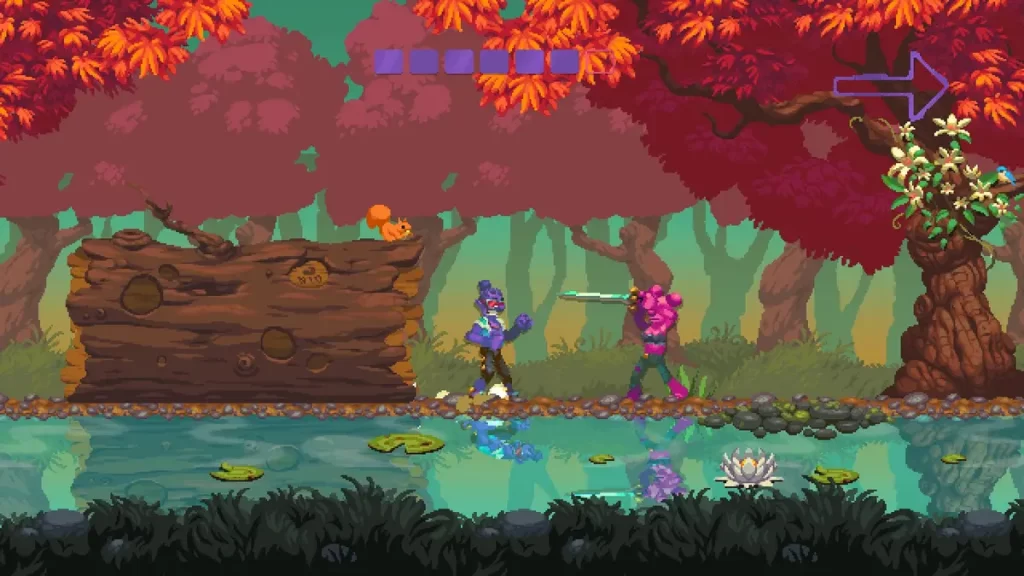
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
public class GamePanel extends JPanel implements KeyListener {
private int x = 50; // Initial player position
private int y = 50;
public GamePanel() {
addKeyListener(this);
setFocusable(true);
}
@Override
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if (key == KeyEvent.VK_LEFT) {
x -= 10; // Move left
} else if (key == KeyEvent.VK_RIGHT) {
x += 10; // Move right
}
repaint(); // Refresh the panel to update the character's position
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.fillRect(x, y, 30, 30); // Draw player as a rectangle
}
// Unused methods
@Override
public void keyTyped(KeyEvent e) {}
@Override
public void keyReleased(KeyEvent e) {}
}
Instead of basic shapes, you can use sprites – images representing characters, objects, and backgrounds. Java’s BufferedImage
class is useful for loading and drawing images on the game canvas. For animations, you can create a sequence of images and change them rapidly to simulate motion.
BufferedImage playerSprite = ImageIO.read(new File("player.png"));
g.drawImage(playerSprite, x, y, this);
And then you need to create a game loop to ensure that the game updates and renders continuously, creating smooth motion and interactions. The loop typically handles updating game logic, processing user input, and rendering the game screen.
while (gameRunning) {
update(); // Update game logic
render(); // Render the game
Thread.sleep(16); // Maintain 60 FPS
}
Step 4: test, optimize, and share your game
Testing is crucial in game development. Run your game frequently to catch bugs and optimize performance. If the game slows down, consider optimizing graphics rendering or tweaking your code to reduce unnecessary computations.
Also, if possible, develop a group of alpha and beta playtesters. Once your game is complete, you can export it as a standalone application. In Java, this usually involves packaging the game into a .jar (Java ARchive) file. You can also use Java Web Start for web-based game distribution.
How to develop games in Java with professionals
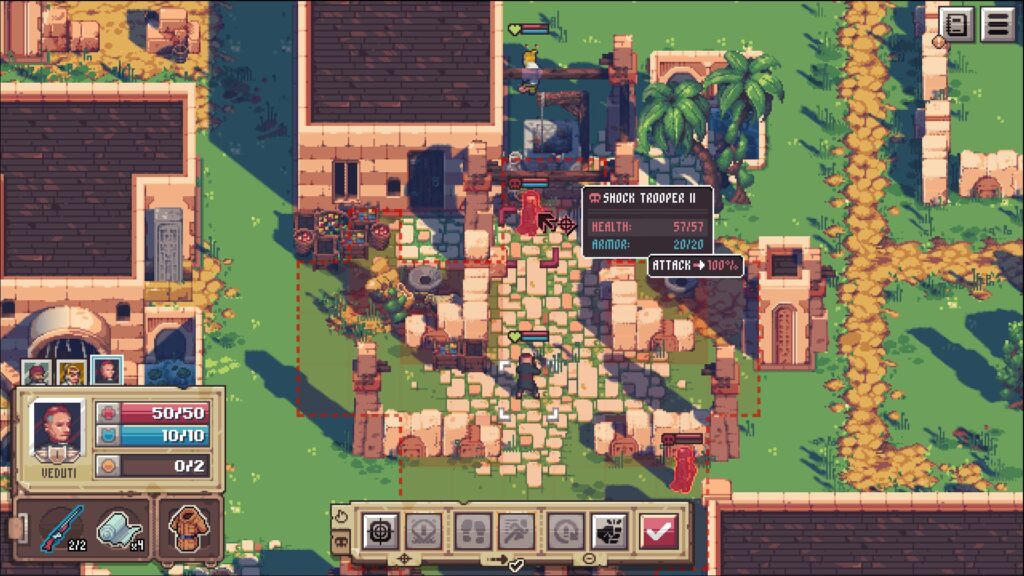
Developing games in Java is a rewarding experience that opens up endless creative possibilities all in all. By following these steps, you’ll be well on your way to creating your own games. With Java’s extensive libraries and frameworks, you can start small and gradually build more complex and engaging games.
But, of course, if you have the opportunity, working with professionals in the field is always a great idea when developing a game. So, if you need help with the development of games in Java, you can always contact us here at Main Leaf.
We would love to put our 12 years of experience in the world of video game development at your service and help you bring your idea to life. Get in touch with us right now to learn more about our work!
Also, if you liked this article, don’t forget to check our blog, where we discuss many other topics relating to the world of video games and video game development.