Ask anyone who dreams of working in the game industry, chances are they have at least considered working with coding. The realm of game development offers you different paths to manifest your creativity, but learning how to code a game is perhaps the most engaging and interactive way possible.
Learning how to code a game not only unveils the magic behind your favorite digital worlds but also empowers you to craft something exceptionally distinctive.
In this comprehensive article, we embark on an exploration of the landscape of game coding, traversing through some of the industry’s most renowned programming languages.
As we embark on this journey, we will be accompanied by illustrative code examples that will serve as your guiding light to step confidently into the realm of game development.
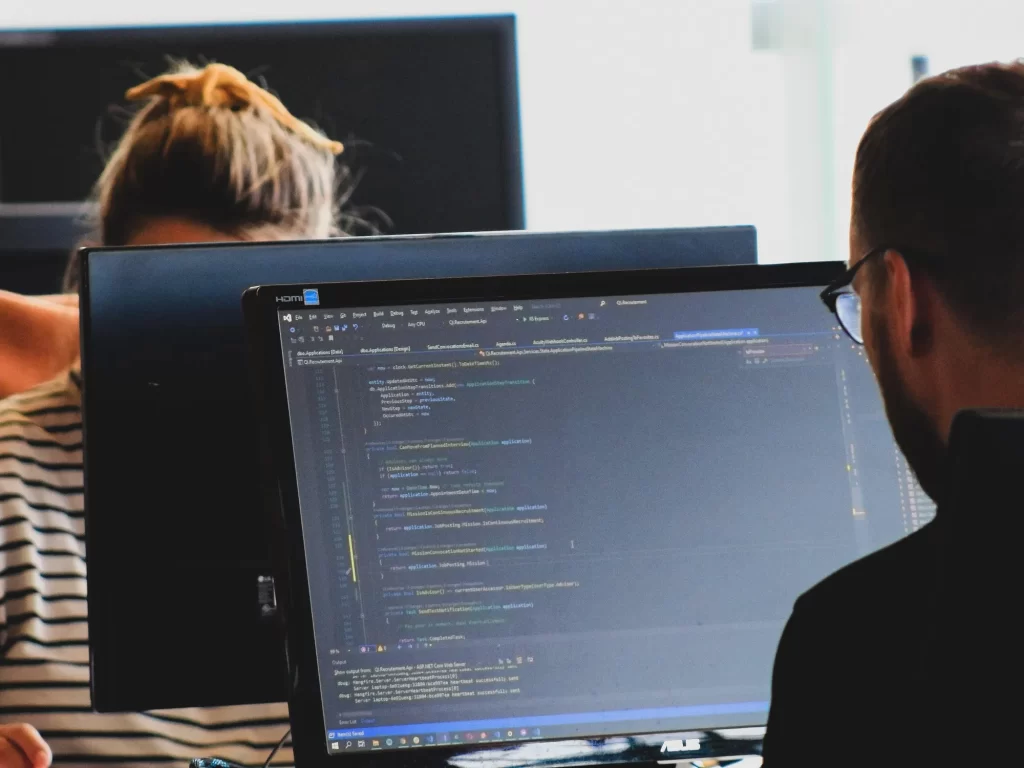
How to code a game: first steps
Before delving into how to code a game, there are several essential concepts and skills you should familiarize yourself with to ensure a smoother development process and a successful end product.
Understanding basic programming concepts like variables, data types, conditional statements, loops, and functions is pivotal. So start by checking if you’re familiar with all these concepts.
Brush up on mathematics, especially algebra and geometry. These are crucial for tasks like collision detection, physics simulations, and character movement.
Study the basics of 2D and 3D graphics concepts, including pixels, vectors, textures, and shaders. This is important for creating a kind of “proto-design” that will help you visualize what you are coding.
Studying game design principles such as player motivation, game loops, balancing, and player engagement can help a ton when learning how to code a game.
If your game involves physics simulations, studying concepts like gravity, collisions, and rigid bodies can also help tremendously.
But most important of all is to work on your patience and persistence. Game development can be challenging, so cultivating patience and persistence to overcome obstacles and see your project through is a very important step.
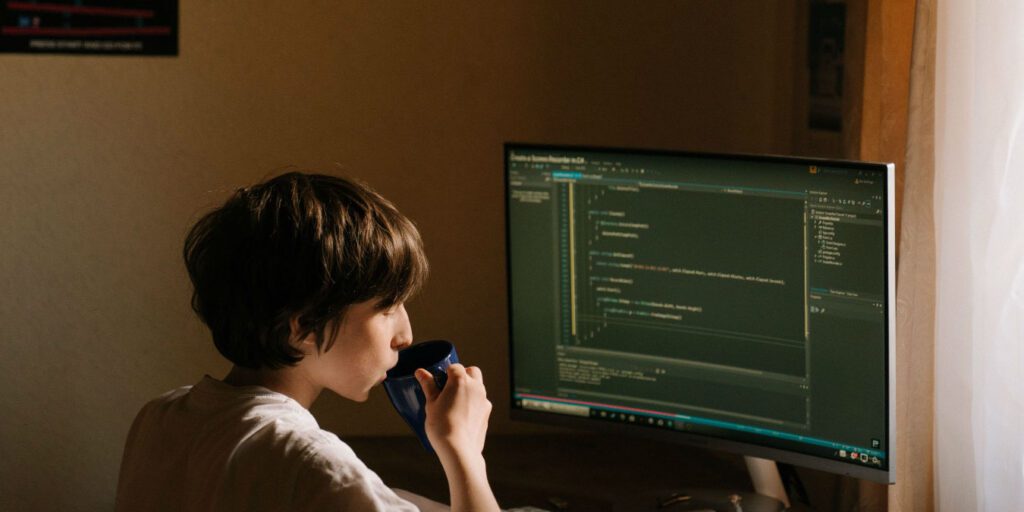
Most popular coding languages
Learning how to code a game can be a completely different experience depending on what platform you are going to choose to work on.
In this article, we are going to focus on the three most popular programming languages today: Python, JavaScript, and C#.
So let’s talk about each one separately and give you some examples of simple codes in each platform.
Python
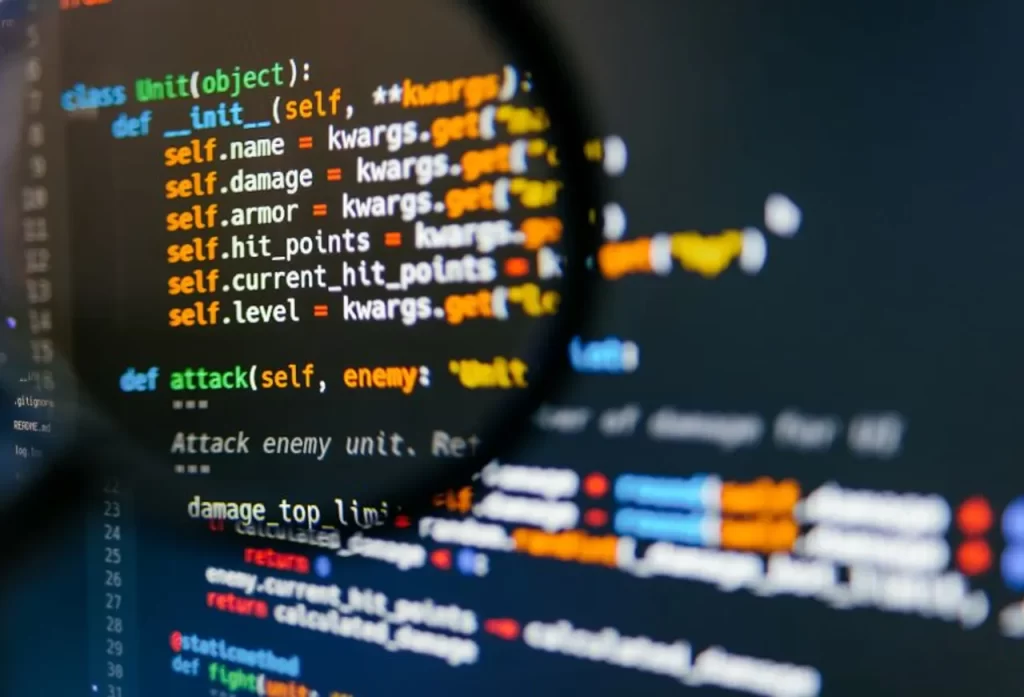
Python stands out in the realm of programming languages due to its reputation for simplicity, elegance, and readability, all of which contribute to making it an exceptional choice, especially for those venturing into the world of coding for the first time.
Its human-friendly syntax allows beginners to focus on understanding fundamental programming concepts without getting bogged down by intricate language intricacies.
Python’s versatility extends well beyond introductory programming. Its vast ecosystem of libraries and frameworks caters to a wide array of applications, and one standout example in the realm of game development is Pygame.
Pygame, a popular Python library, empowers aspiring game developers to bring their creative visions to life by providing a robust set of tools and functionalities.
It’s particularly advantageous for beginners due to its well-documented and user-friendly nature. With Pygame, creating a basic game becomes an accessible endeavor even for those with limited coding experience.
The following code is an example of a simple pong game created using Python.
import pygame
import sys
# Initialize Pygame
pygame.init()
# Set up the game window
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Pong Game")
# Game loop
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Clear the screen
screen.fill((0, 0, 0))
# Update the display
pygame.display.update()
JavaScript
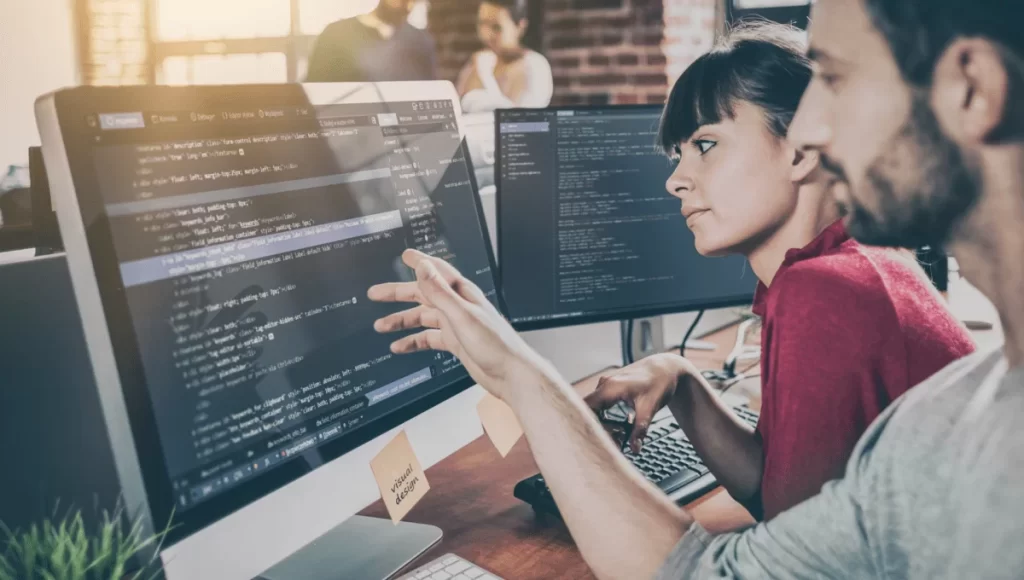
Ever since its first conception, JavaScript has emerged as a powerhouse in the realm of web-based game development, leveraging its unique ability to run directly within web browsers.
This capability has revolutionized the way how to code a game and how they are delivered and experienced, opening up new possibilities for interactive and engaging experiences on the web.
At the heart of this transformation lies the HTML5 “canvas” element, a versatile tool that serves as a dynamic drawing area for rendering graphics in real time.
It essentially acts as a blank slate where developers can paint graphics, animations, and interactive elements using JavaScript code.
This approach allows for precise control over every pixel, making it an excellent choice for game designers seeking to craft unique visual experiences.
Here is an example of a code in JavaScript for a classic brick breaker game.
const canvas = document.getElementById("gameCanvas");
const ctx = canvas.getContext("2d");
// Set up game objects
const ball = { x: canvas.width / 2, y: canvas.height - 30, dx: 2, dy: -2, radius: 10 };
const paddle = { x: canvas.width / 2 - 50, y: canvas.height - 10, width: 100, height: 10 };
// Game loop
function draw() {
// Clear the canvas
ctx.clearRect(0, 0, canvas.width, canvas.height);
// Draw ball
ctx.beginPath();
ctx.arc(ball.x, ball.y, ball.radius, 0, Math.PI * 2);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
// Draw paddle
ctx.beginPath();
ctx.rect(paddle.x, paddle.y, paddle.width, paddle.height);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
// Update ball position
ball.x += ball.dx;
ball.y += ball.dy;
requestAnimationFrame(draw);
}
draw();
C#
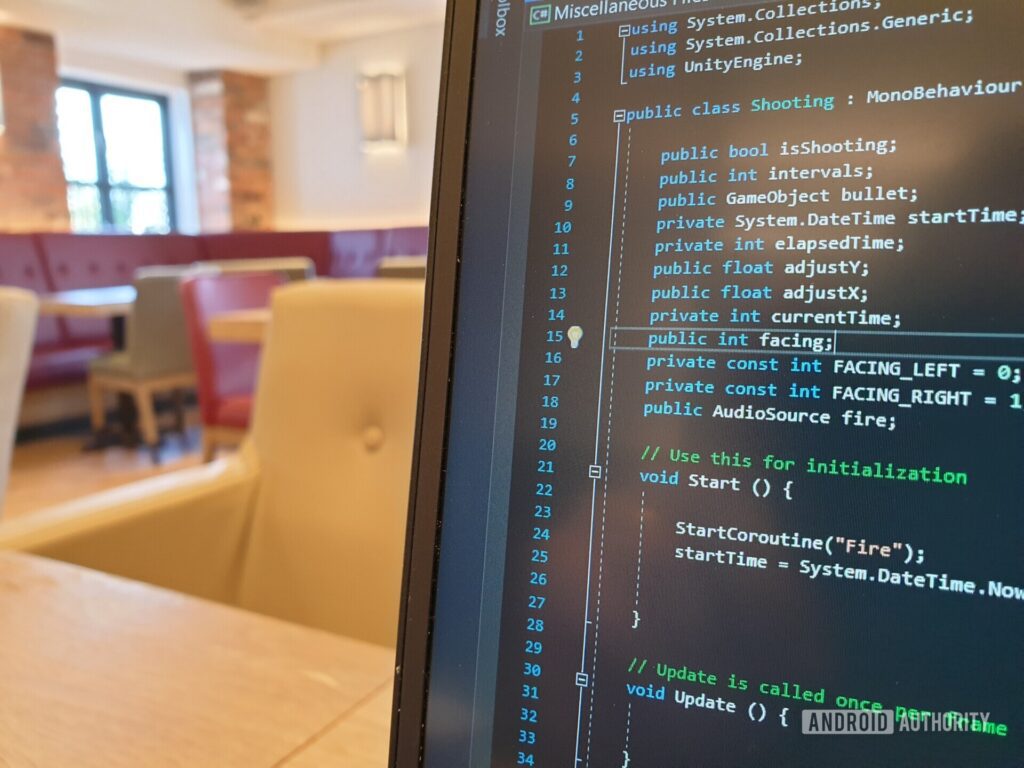
C# stands as a versatile and powerful programming language that finds application across a wide spectrum of software development, and it is an important language when it comes to how to code a game.
Within the realm of game development, it serves as a foundational language for creating interactive and immersive experiences, particularly when combined with the Unity game engine.
C# boasts a balance between high-level and low-level programming, making it versatile enough to handle both complex game mechanics and efficient memory management.
This versatility allows game developers to write code that meets the performance demands of modern games, but also keep things simple if they are still learning the intricacies of game coding.
The Unity game engine, a leader in the industry, relies heavily on C# for scripting. Unity provides a user-friendly and visually-oriented environment for building games, and C# is the language used to control gameplay behavior, create interactions, manage assets, and implement game mechanics.
Here is an example of how you would code character movement in a 2D game created using Unity.
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed = 5f;
public float jumpForce = 10f;
private Rigidbody2D rb;
private bool isGrounded;
void Start()
{
rb = GetComponent<Rigidbody2D>();
}
void Update()
{
float moveX = Input.GetAxis("Horizontal");
rb.velocity = new Vector2(moveX * speed, rb.velocity.y);
if (Input.GetButtonDown("Jump") && isGrounded)
{
rb.AddForce(Vector2.up * jumpForce, ForceMode2D.Impulse);
isGrounded = false;
}
}
void OnCollisionEnter2D(Collision2D collision)
{
if (collision.gameObject.CompareTag("Ground"))
{
isGrounded = true;
}
}
}
Start learning how to code a game today
Coding a game can be a fantastic journey that combines creativity and technical skills. Whether you choose Python, JavaScript, C#, or any other programming language, the key is to start with small projects and gradually work your way up to more complex games.
As you gain experience, you’ll find that game development becomes increasingly enjoyable and satisfying. So, pick a language, grab your favorite game idea, and start coding your very own game today!
We here at Main Leaf, over our 11 years in the game development market, have experimented with many languages, and we know firsthand that practice makes perfect.
Since we work with Unity and Unreal, C+ is often our language of choice – but we acknowledge the potential of other languages, and we know that what makes a good code is the coder, not the platform.
If you liked this article, check out our blog for many more articles related to the game development world. Or, if you have a project in mind, don’t hesitate to contact us so we can help develop it. Get to know our services – we’ll answer you within 24 hours.